Excerpt
In this article, you will learn how to use an Arduino board and a button to control an LED. The article provides a step-by-step guide to setting up the circuit and programming the board using the Arduino IDE. The code example demonstrates how to turn on the LED when the button is pressed and turn…
Description
In this course, you will learn how to use a button to control an LED on an Arduino board. You will start by understanding the basic concepts of Arduino programming and circuitry, and then move on to learning how to set up the circuit and write the code to turn the LED on and off using the button. You will also learn how to use conditional statements and functions to create more complex behaviors for your LED. By the end of this course, you will have a solid understanding of how to use buttons to control LEDs on your Arduino, and you’ll be ready to create your own interactive projects! Get ready to unleash your inner inventor and join us on this fun and engaging Arduino adventure!
To complete the project of using a button to switch an LED on and off on an Arduino, the following components and tools will be required:
- Arduino board (e.g., Arduino Uno)
- Breadboard
- LED (any color)
- 220 Ohm resistor
- Push button switch
- Jumper wires
- USB cable (Type A to Type B)
These components can typically be found in most electronics starter kits, and can also be purchased individually online or at electronics stores. It’s important to make sure that the LED, resistor, and push button switch are all rated for use with the Arduino’s voltage and current limits. Additionally, a computer with the Arduino IDE (Integrated Development Environment) installed will be needed to write and upload the code to the Arduino board.
Setting up the Circuit
To set up the circuit for controlling an LED using a button with Arduino, follow these steps:
- Gather the required components and tools, as listed in the previous section.
- Connect one end of the resistor to the long leg of the LED. The resistor should have a value of around 220 ohms.
- Connect the other end of the resistor to an empty row on the breadboard.
- Connect the short leg of the LED to the negative rail on the breadboard.
- Connect one leg of the button to an empty row on the breadboard.
- Connect the other leg of the button to the negative rail on the breadboard.
- Connect a wire from the row with the button to digital pin 2 on the Arduino.
- Connect a wire from the row with the LED to digital pin 3 on the Arduino.
- Finally, connect the Arduino to your computer using a USB cable.
The circuit is now set up and ready to be programmed.
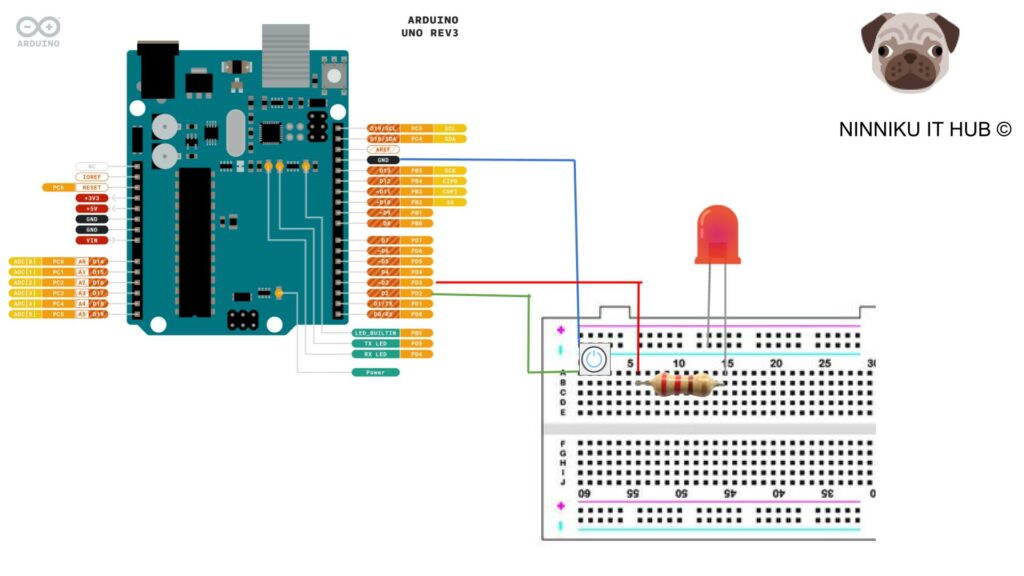
Preventing Floating State
When using digital pins as inputs in Arduino, it is easy to encounter a floating state. Normally, a resistor pull-up or pull-down is used to solve this problem. In this example, we use the built-in resistor pull-up in Arduino to simplify the circuit complexity.
If you want to understand what Pull-up and Pull-down are, you can refer to the following article.
Programming the Arduino Board
Now that the circuit is set up, it’s time to write the code that will control the LED and read the button input. The Arduino programming language is based on C++, and the Arduino IDE provides a simplified programming environment with built-in functions and libraries that can be used to interact with the board’s hardware.
Here’s an example code that will turn on the LED when the button is pressed, and turn it off when the button is released:
// Pin assignments
const int buttonPin = 2;
const int ledPin = 3;
// Variables
int buttonState = 0;
void setup() {
pinMode(buttonPin, INPUT_PULLUP);
pinMode(ledPin, OUTPUT);
Serial.begin(9600);
}
void loop() {
// Read the state of the button
buttonState = digitalRead(buttonPin);
Serial.print("buttonPin value: "); // Print potentiometer value
Serial.print(buttonPin);
Serial.print(" Status: ");
Serial.println (buttonState);
// If the button is pressed, turn on the LED
if (buttonState == LOW) {
digitalWrite(ledPin, HIGH);
}
// Otherwise, turn off the LED
else {
digitalWrite(ledPin, LOW);
}
}
The code starts by defining the pin numbers for the button and LED using const int
variables. In the setup()
function, we set the button pin as an input (pull-up) and the LED pin as an output using the pinMode()
function.
In the loop()
function, we read the state of the button using digitalRead()
and store it in the buttonState
variable. If the button is pressed (buttonState
is HIGH
), we turn on the LED using digitalWrite()
. Otherwise, we turn off the LED by setting the LED pin to LOW
.
You can customize this code to change the pin assignments or add more functionality, such as changing the LED brightness based on how long the button is pressed. Once you’re done editing the code, you can upload it to the Arduino board by clicking the “Upload” button in the IDE. The code will be compiled and transferred to the board’s microcontroller, and the LED and button should start responding to the button presses.
Customizing and Expanding the Circuit
Once you have successfully programmed the Arduino board to control the LED using a button, you can start customizing and expanding the circuit. Here are some ideas to get you started:
- Add more LEDs: You can add multiple LEDs to the circuit and control them with different buttons. This can create more complex lighting effects.
- Use different types of buttons: Experiment with different types of buttons, such as push buttons, toggle switches, or capacitive touch sensors. Each type of button will require different wiring and programming.
- Integrate with other sensors: You can add other sensors to the circuit, such as light sensors or motion sensors, to create a more interactive and responsive system.
- Connect to the internet: If you have an internet-connected Arduino board, you can control the LED and button remotely using a smartphone or computer.
- Build a custom enclosure: Once you have finalized your circuit, you can build a custom enclosure to house the Arduino board, the LED, and the button. This will give your project a more polished and professional look.
Remember, the possibilities are endless when it comes to customizing and expanding your Arduino circuit. Don’t be afraid to experiment and try new things.
Conclusion
In conclusion, building a button-controlled LED circuit on an Arduino board is a fun and educational project that can be completed by beginners and advanced hobbyists alike. With a few basic components and tools, anyone can create a working circuit and customize it to their liking. By following the steps outlined in this guide, you can learn the basics of electronics and programming while creating a fun and interactive project. So go ahead and try it out, and see what other cool things you can create with your Arduino board!